Why use Repository Pattern in Laravel
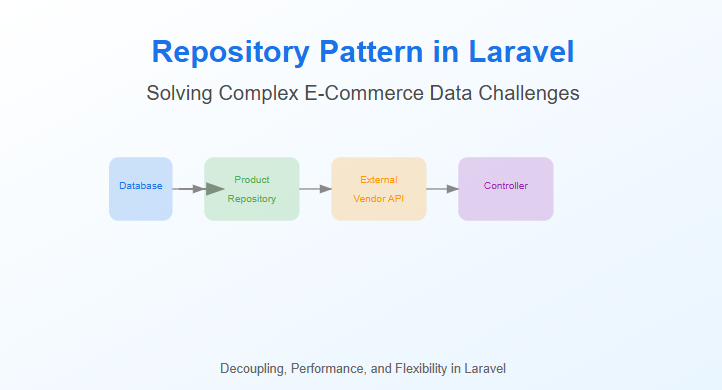
Detailed Use Case: E-Commerce Platform with Complex Data Requirements
Scenario: Multi-Vendor Marketplace System
Initial Context
Imagine an e-commerce platform with the following complex requirements:
– Multiple data sources (internal database, external vendor APIs)
– Complex querying across different models
– Need for extensive caching
– Requirement for advanced filtering and search
– Performance optimization
– Decoupled architecture for scalability
Practical Implementation
- Product Repository with Multiple Data Sources
namespace App\Repositories;
use App\Models\Product;
use App\Interfaces\ProductRepositoryInterface;
use Illuminate\Support\Facades\Cache;
use App\Services\ExternalVendorService;
class ProductRepository implements ProductRepositoryInterface
{
protected $model;
protected $externalVendorService;
public function __construct(Product $product, ExternalVendorService $externalVendorService)
{
$this->model = $product;
$this->externalVendorService = $externalVendorService;
}
// Complex method demonstrating multiple concerns
public function getFilteredProducts(array $filters)
{
// Caching strategy
$cacheKey = $this->generateCacheKey($filters);
return Cache::remember($cacheKey, now()->addHours(2), function () use ($filters) {
$query = $this->model->newQuery();
// Dynamic filtering with multiple conditions
$query->when($filters['category'] ?? null, function ($q, $category) {
return $q->where('category_id', $category);
})
->when($filters['price_min'] ?? null, function ($q, $minPrice) {
return $q->where('price', '>=', $minPrice);
})
->when($filters['price_max'] ?? null, function ($q, $maxPrice) {
return $q->where('price', '<=', $maxPrice);
})
->when($filters['vendor'] ?? null, function ($q, $vendor) {
return $q->where('vendor_id', $vendor);
});
// Complex join with vendor information
$query->with(['vendor', 'reviews'])
->withCount('reviews')
->orderBy('rating', 'desc');
// Paginate with custom per page
return $query->paginate($filters['per_page'] ?? 15);
});
}
// Method to fetch products from external vendor
public function getExternalVendorProducts($vendorId)
{
// Fetch products from external API
$externalProducts = $this->externalVendorService->getProducts($vendorId);
// Transform and normalize external data
return $this->transformExternalProducts($externalProducts);
}
// Advanced search method
public function searchProducts($searchTerm, array $options = [])
{
return $this->model->search($searchTerm)
->when($options['category'] ?? null, function ($query, $category) {
return $query->where('category_id', $category);
})
->when($options['vendor'] ?? null, function ($query, $vendor) {
return $query->where('vendor_id', $vendor);
})
->paginate($options['per_page'] ?? 15);
}
// Complex aggregation method
public function getVendorProductPerformance($vendorId)
{
return $this->model->where('vendor_id', $vendorId)
->select([
DB::raw('AVG(rating) as average_rating'),
DB::raw('COUNT(*) as total_products'),
DB::raw('SUM(total_sales) as cumulative_sales')
])
->first();
}
// Private helper method for cache key generation
private function generateCacheKey(array $filters)
{
return 'products:' . md5(json_encode($filters));
}
}
Why Repository Pattern is Necessary Here
- Separation of Concerns
– Isolates complex database logic from controllers
– Centralizes query logic in one place
– Makes code more maintainable and readable
- Flexibility in Data Sources
– Can easily switch between local database and external APIs
– Provides a consistent interface for data retrieval
– Allows seamless integration of multiple data sources
- Caching Strategy
– Implements sophisticated caching mechanism
– Reduces database load
– Improves application performance
- Complex Filtering and Search
– Provides advanced filtering capabilities
– Supports dynamic query building
– Enables complex search across multiple criteria
- Testability
// Easy to mock for unit testing
public function testProductRetrieval()
{
$mockRepo = Mockery::mock(ProductRepository::class);
$mockRepo->shouldReceive('getFilteredProducts')
->once()
->andReturn(collect([]));
// Test controller or service logic
}
Additional Benefits in This Scenario
– Performance Optimization
– Centralized query optimization
– Consistent caching strategy
– Reduced database hits
– Scalability
– Easy to modify data retrieval logic
– Supports future extensions
– Decoupled from direct Eloquent usage
When This Approach Shines
👉 Large, complex applications
👉 Multiple data sources
👉 Advanced querying requirements
👉 Performance-critical systems
👉 Microservices architectures
Potential Drawbacks (Mitigated)
- Complexity: Managed through clean, well-structured implementation
- Performance Overhead: Minimized through efficient caching
- Abstraction: Provides tangible benefits in complex scenarios
Key Takeaway
The Repository pattern is not about blind abstraction but strategic decoupling. In scenarios with complex data interactions, it provides significant architectural advantages that outweigh its minor performance implications.